Welcome to Composio
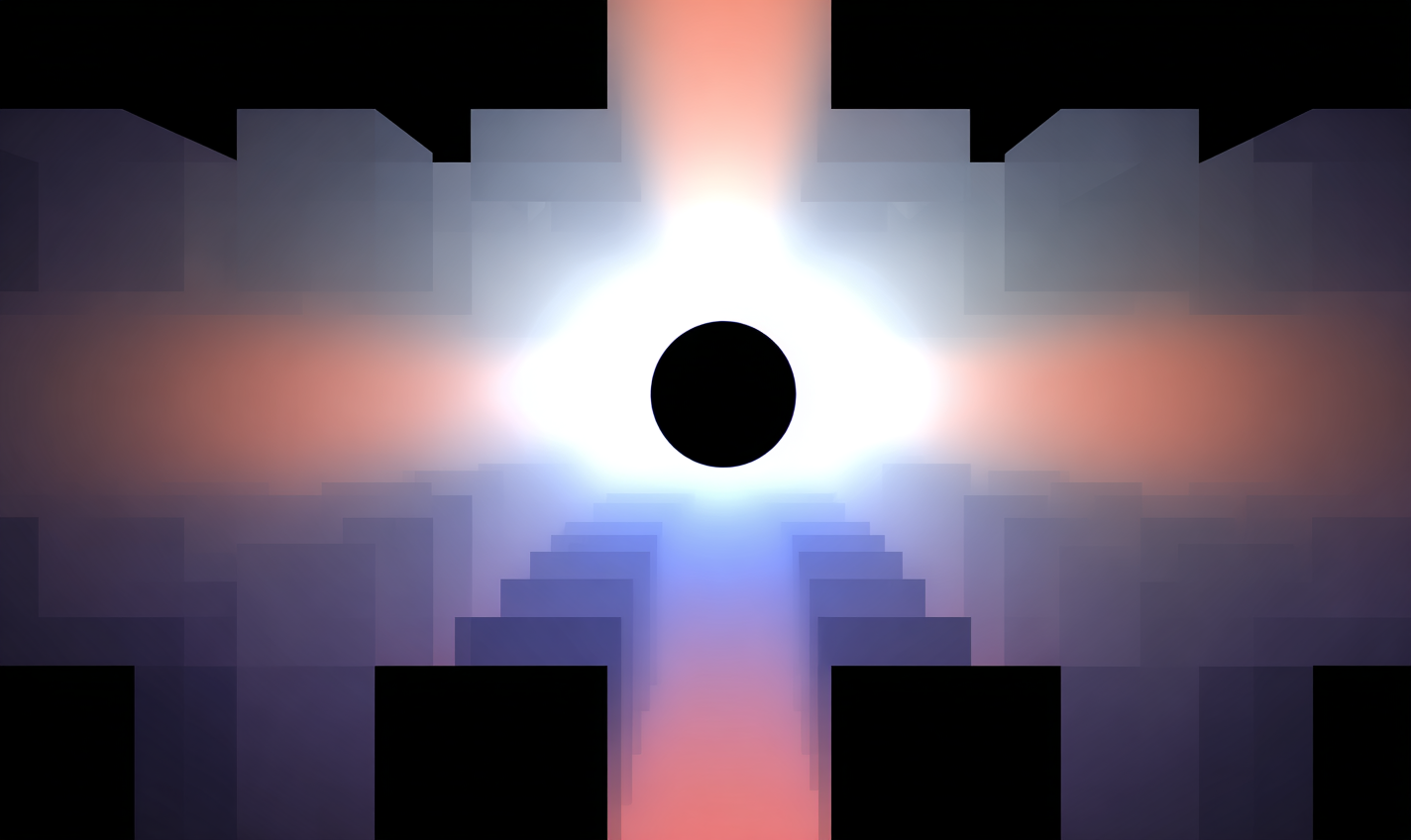
Give your AI IDE access to Composio’s documentation using our llms.txt files: (short .txt, long .txt)
Composio is the fastest way to enable your AI agents to take real-world actions—without dealing with individual API integrations, authentication flows, or complex tool formatting.
- Access 3000+ tools out of the box across popular apps like Slack, GitHub, Notion, and more. Browse tools →
- Enforce strict access and data controls with fine-grained permissions for each tool and user.
- Trigger agents and workflows using external events (e.g., new message in Slack, new issue in GitHub).
- Customize tools for your proprietary APIs or internal functions via modifiers.
- Integrate seamlessly with frameworks like OpenAI, Anthropic, LangChain, Vercel AI SDK, and more.
- Enhance reliability with input/output processing hooks to clean or transform data on the fly.
No more writing dozens of bespoke integrations. Composio handles auth, tool normalization, and execution—so your agents can focus on reasoning, not plumbing.
We even optimize tool calls automatically for better accuracy—free of cost.