White-labelling
Guide to white-labelling the OAuth Flow
Using custom auth app (aka white-labelling)
When going to production, it’s recommended to use your own developer credentials.
Set up a 301 redirect
The endpoint https://backend.composio.dev/api/v1/auth-apps/add
is what captures the user’s credentials to manage the auth. However, OAuth consent screens show the callback URL - and if it isn’t the same as your application, that creates distrust.
It’s recommended to specify the redirect URL to your own domain and create a redirect logic, either through your DNS or in your application to redirect that endpoint to https://backend.composio.dev/api/v1/auth-apps/add
Setting up a redirect through DNS
This diagram shows the entire redirect sequence.
Create the integration
Create your integration, specifying the redirect URL in the auth configuration.
use_composio_oauth_app
/ useComposioAuth
flag to False!Refer to the concepts page for more information on how to retrieve the auth configuration for an integration.
The connection request returns a redirect URL that you can emit to the user to start the auth process. They see the custom consent screen that you configured. In this case, it’s “usefulagents.com”
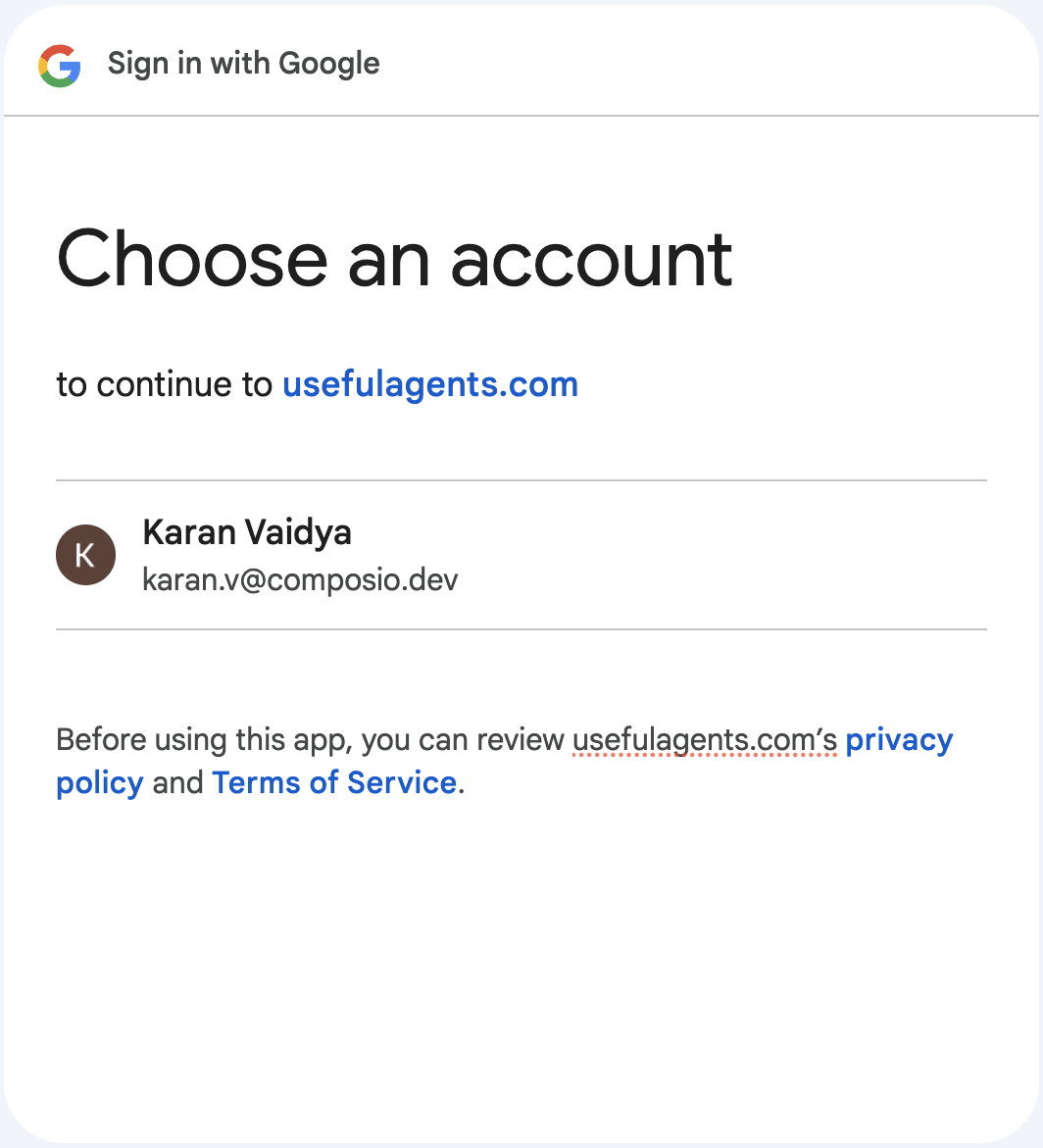