Auth Concepts
Understand the core concepts behind Composio Auth
Composio Auth simplifies integrating user-authorized actions into your app. It securely manages OAuth flows, handles token storage, and ensures actions are executed with the correct user’s credentials.
Composio Auth relies on three core concepts. Integration, Connection, Entities.
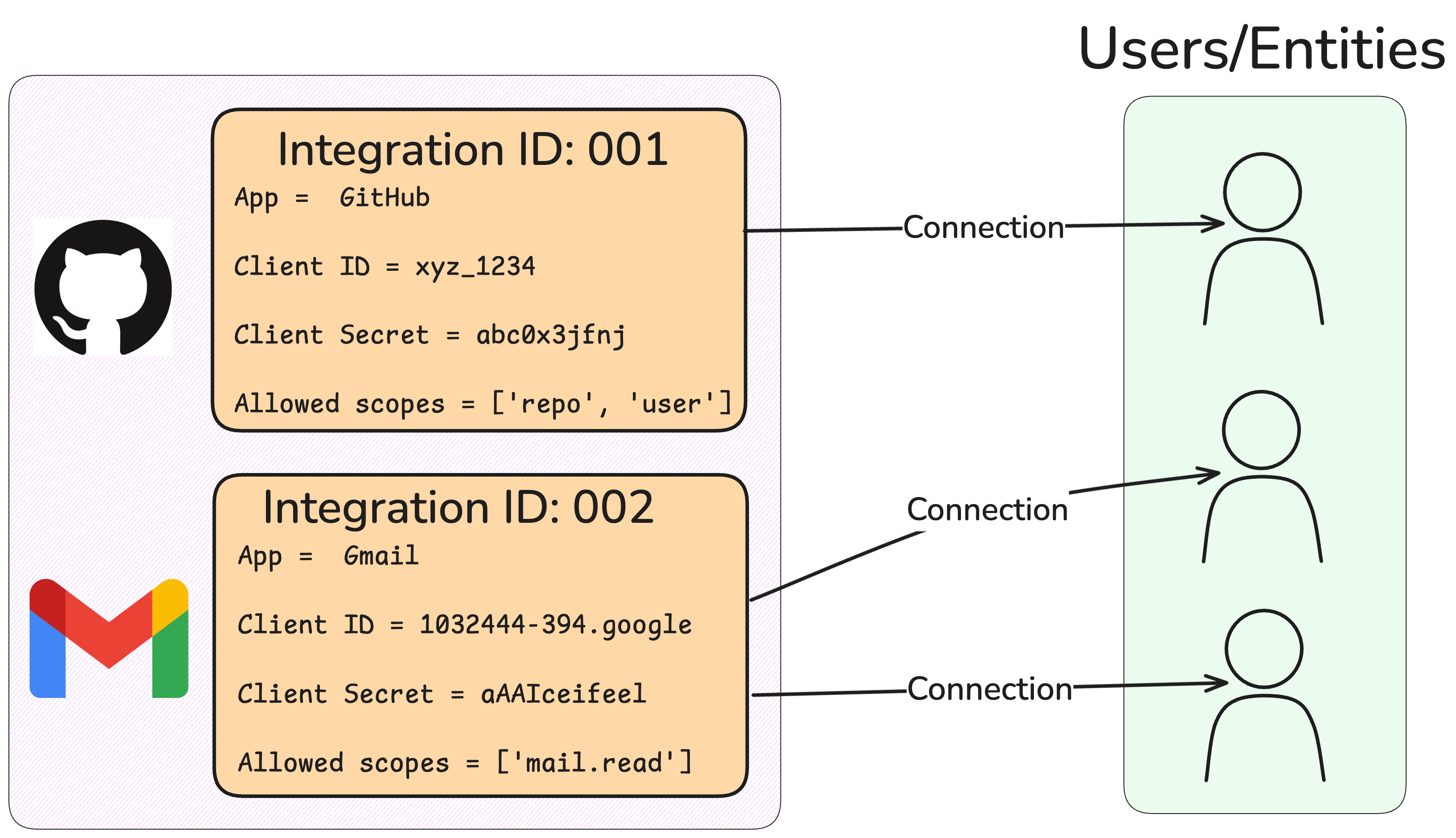
About authentication and authorization
Authentication means checking that a user is who they say they are. This is about establishing WHO the user is.
Authentication Flow
For example, when a user connects their Gmail account to your app, Composio Auth guides the user through Google’s OAuth login. The user securely proves their identity to Google, and Composio safely stores the resulting access token, confirming the user’s identity without you handling sensitive credentials.
On expiry, Composio Auth automatically refreshes the access token, ensuring continuous, secure access without requiring users to re-authenticate.
Authorization means checking what resources a user can access. This is about controlling WHAT the authenticated user can do.
Authorization Flow
Continuing the Gmail example, authorization happens when your app attempts to access a user’s inbox. Scopes are used to control what resources a user can access. The application controls access to the resources based on the scopes requested during authentication.
Explained below are some authentication schemes that Composio supports.
OAuth 2.0
OAuth 2.0 allows users to securely grant limited permissions to your app for accessing their data on third-party services without sharing actual login credentials.
Composio Auth streamlines OAuth by managing user authorization, token handling, secure storage, and token refreshing.
OAuth 2.0 Flow:
OAuth uses Scopes to define the exact permissions your app needs (e.g., mail.read
for Gmail). Composio helps ensure secure, precise configuration of scopes.
API Key
API Keys are simple credentials used to directly access services, usually with broad access privileges.
Composio securely stores API keys, automatically applying them to API requests, providing secure yet straightforward access.
API Key Flow:
Bearer Token
Bearer Tokens are credentials sent in HTTP headers to securely access protected resources without needing underlying user credentials.
Composio securely manages Bearer Tokens, embedding them in API requests automatically, simplifying secure token handling.
Bearer Token Flow:
Basic Authentication
Basic Authentication encodes and sends user credentials (username and password) with each request.
Composio securely encodes and handles these credentials, managing headers securely, ensuring sensitive data protection.
Basic Authentication Flow:
JSON Web Token (JWT)
JWT is a compact, secure method for encoding user information, claims, and permissions for safe transmission between systems.
Composio manages JWT lifecycle, including creation, validation, verification of signatures, expiration handling, and secure storage.
JWT Flow:
Integration
An Integration in Composio is a configuration that connects your app to third-party services like GitHub, Google Drive, or Slack. It contains all the authentication details (OAuth 2.0, API Key, or JWT) and service-specific settings needed to create secure connections for your users.
Essentially, for each third-party service, you only need one integration that manages multiple users.
Integration Properties
An Integration stores all necessary authentication and API communication details required for making authenticated requests to third-party services. These properties define how Composio interacts with the external API.
Authentication Methods
Composio supports multiple authentication schemes, and each Integration can define one or more of these:
- Developers configure Integrations once per third-party service.
- Users authenticate against these Integrations to establish personalized connections.
- API calls are routed through Composio, leveraging stored authentication details for seamless execution.
Creating Integrations
Code
Dashboard
CLI
Below code snippet some helper functions while working with integrations.
(Optional) Specifying Client Credentials
For OAuth 2.0 integrations where it is recommended to provide your own client credentials read: OAuth Client Credentials, you can specify your client credentials while creating the integration.
First view the expected params
Expected Param Object
Below is the full code to creating and managing an integration!
Full Code
Entity
An Entity in Composio serves as an abstraction over a user in your application. It represents an individual user and provides a structured way to manage their authenticated API interactions.
Instead of directly handling user authentication at the application level, Composio uses Entities to link users to their respective service connections.
-
User Identification: Entities allow you to manage users in Composio without directly dealing with user-specific authentication details.
-
Seamless Authentication: Each Entity can have multiple connections to third-party services, enabling secure API interactions on behalf of users.
-
Scoped API Execution: When executing API calls, the Entity ID ensures Composio applies the correct user’s authentication details.
Entity Management
Entities provide a set of methods that allow you to manage connections for each user efficiently.
Once you create the Entity object, you can use it to handle tool calls and execute actions. Alternatively, you can specify the Entity ID itself while handling tool calls.
In essence, an Entity in Composio is the mechanism that links your application’s user identity system with Composio’s connection management, enabling personalized API interactions on behalf of specific users.
Connection
A Connection in Composio represents an authenticated link between a specific entity and an external service through an Integration.
An integration can have multiple connections, each representing a different user’s authentication state.
It’s an abstraction that stores and manages your users’ auth configuration. Connections serve as the runtime authentication context that enables Composio to:
- Make authenticated API calls on behalf of specific users.
- Automatically refresh tokens before expiry.
- Associate API responses with the correct user.
Creating Connections
To create a connection for an entity, you need to provide the auth scheme and the connection parameters.
Creating a connection in application level code requires some basic steps.
Fetch Integration ID and Entity
You need to fetch the entity_id
from your database or application logic.
Retrieve connection parameters
Code
Dashboard
Connection parameters vary per integration. For example, OAUTH2
based integrations don’t require any additional parameters from the user, however other methods may require things like the Base URL or JWT token.
Fetching connection parameters with an integration ID
Fetching connection parameters with app and auth scheme
Examples
Connection with OAuth 2.0
OAuth 2.0 applications generally don’t have any expected_params
since the OAuth flow is supposed to capture and store the relevant credentials.
Once the connection object has been created, the redirectUrl
has to be emitted back to the user so that they can follow the OAuth credentials.
Connection with Bearer Token
Many applications that have OAuth 2.0, also support the user providing their own Bearer token.
Connection with API Key
You can ask the user’s to enter in their API key for apps and services that support it. Composio will store the key securely.